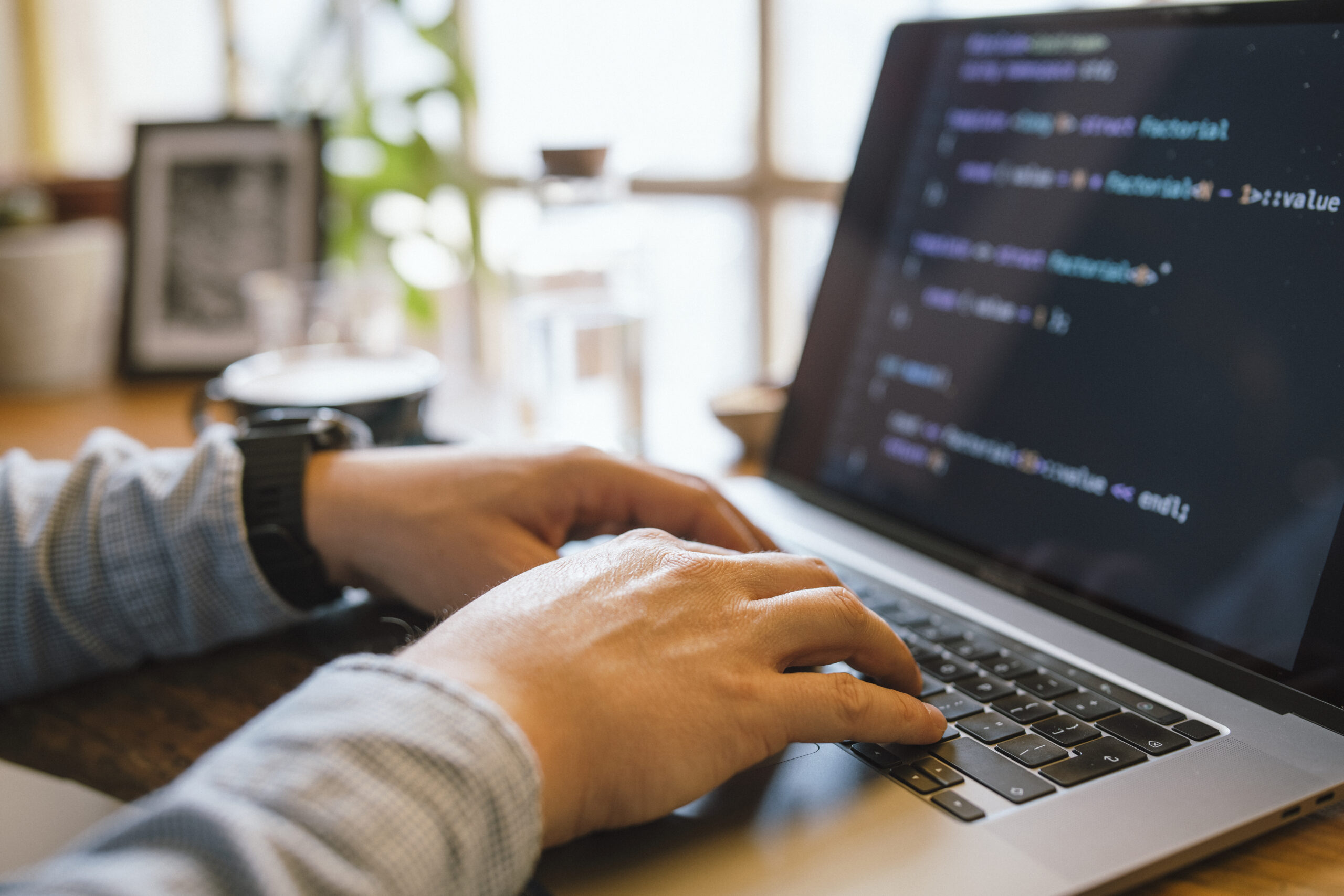
Debugging is Just about the most vital — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Assume methodically to unravel challenges successfully. Irrespective of whether you are a rookie or simply a seasoned developer, sharpening your debugging techniques can help you save several hours of annoyance and considerably transform your productiveness. Allow me to share many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of many quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst crafting code is a person Section of growth, being aware of the way to interact with it successfully during execution is Similarly critical. Present day advancement environments come equipped with highly effective debugging capabilities — but a lot of developers only scratch the surface area of what these tools can perform.
Acquire, for example, an Built-in Growth Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and perhaps modify code within the fly. When used effectively, they Enable you to observe accurately how your code behaves for the duration of execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into workable responsibilities.
For backend or program-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command systems like Git to comprehend code heritage, obtain the precise instant bugs have been launched, and isolate problematic variations.
Finally, mastering your tools indicates heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when challenges crop up, you’re not lost in the dark. The better you know your tools, the greater time you could expend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most essential — and sometimes ignored — actions in effective debugging is reproducing the problem. Right before leaping to the code or creating guesses, developers have to have to produce a steady surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you might have, the simpler it results in being to isolate the exact circumstances less than which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the condition in your local natural environment. This could signify inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty may be surroundings-precise — it might occur only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can persistently recreate the bug, you might be now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging resources much more efficiently, check prospective fixes securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract grievance into a concrete challenge — and that’s where builders prosper.
Examine and Recognize the Error Messages
Error messages tend to be the most valuable clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally inform you precisely what happened, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when below time pressure, look at the very first line and immediately start out creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root bring about. Don’t just copy and paste mistake messages into serps — study and have an understanding of them 1st.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date changes within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger concerns and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with realizing what to log and at what level. Typical logging levels include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info throughout development, Facts for normal functions (like profitable start-ups), Alert for likely concerns that don’t break the applying, Mistake for real issues, and Lethal if the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical situations, condition changes, enter/output values, and demanding conclusion factors in your code.
Structure your log messages clearly and continuously. Incorporate context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, wise logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it takes to spot difficulties, gain deeper visibility into your programs, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a specialized undertaking—it is a form of investigation. To efficiently discover and take care of bugs, builders should strategy the method just like a detective resolving a secret. This mindset assists break down sophisticated troubles into workable sections and abide by clues logically to uncover the root cause.
Begin by gathering evidence. Look at the signs of the challenge: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece with each other a transparent image of what’s taking place.
Subsequent, type hypotheses. Inquire oneself: What could possibly be leading to this conduct? Have any adjustments lately been produced to the codebase? Has this difficulty happened ahead of beneath comparable circumstances? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue in a managed surroundings. If you suspect a selected operate or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results direct you nearer to the truth.
Pay near interest to compact information. Bugs frequently cover inside the the very least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and individual, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Finally, retain notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can conserve time for long run issues and aid Many others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical capabilities, approach difficulties methodically, and develop into more effective at uncovering hidden concerns in advanced systems.
Compose Assessments
Producing checks is one of the most effective approaches to transform your debugging competencies and overall improvement effectiveness. Assessments not simply enable capture bugs early but will also function a security Internet that provides you self confidence when building variations to your codebase. A well-tested application is easier to debug because it enables you to pinpoint precisely exactly where and when an issue occurs.
Start with unit tests, which focus on individual capabilities or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as anticipated. Each time a check fails, you instantly know where to look, noticeably lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear right after Formerly being preset.
Upcoming, integrate integration tests and close-to-conclude exams into your workflow. These assist make sure several areas of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in elaborate devices with several factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a function thoroughly, you will need to understand its inputs, anticipated outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that the same bug doesn’t return Later on.
Briefly, writing tests turns debugging from a annoying guessing activity into a structured and predictable method—supporting you capture more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging Gustavo Woltmann coding a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new perspective.
When you're as well close to the code for too lengthy, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into much less effective at problem-resolving. A brief stroll, a coffee break, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the qualifications.
Breaks also assist prevent burnout, Primarily for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power plus a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially underneath tight deadlines, nonetheless it actually contributes to a lot quicker and simpler debugging Ultimately.
In a nutshell, having breaks just isn't an indication of weakness—it’s a sensible technique. It offers your Mind Area to breathe, enhances your perspective, and aids you steer clear of the tunnel eyesight That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Just about every Bug
Every bug you come across is much more than simply A brief setback—It is really an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or simply a deep architectural problem, each can train you a little something valuable in the event you make time to replicate and review what went Incorrect.
Commence by inquiring on your own a handful of key questions after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught previously with superior tactics like device testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving forward.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically potent. Whether it’s via a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team effectiveness and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Every bug you deal with adds a whole new layer towards your skill established. So next time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.